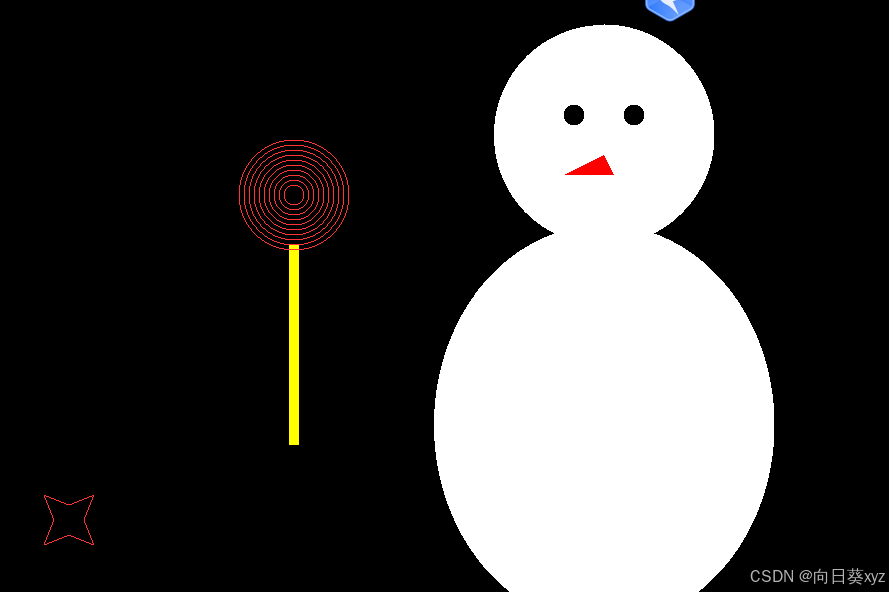
#include <GL/glut.h>
#include <math.h>const int n = 1000;
int q;
int m, p;
const GLfloat R = 0.5f;
const GLfloat Pi = 3.1415926536f;
void init(void)
{glClearColor(0.0f, 0.0f, 0.0f, 0.0f);glShadeModel(GL_FLAT);
}
void DrawCircle()
{int i;glBegin(GL_LINE_LOOP);for (i = 0; i < n; ++i)glVertex2f(m + q * cos(2 * Pi / n * i), p + q * sin(2 * Pi / n * i));glEnd();glFlush();
}
void DrawCirclet1()
{glColor3f(1.0f, 0.0f, 0.0f);GLfloat x, y, z, angle;z = 0.0f;glColor3f(1.0, 1.0, 1.0);glBegin(GL_LINES);for (angle = 0.0f; angle <= Pi; angle += (Pi / 1000000.0f)){x = 610 + 110.0f * sin(angle);y = 460 + 110.0f * cos(angle);glVertex3f(x, y, z);x = 610 + 110.0f * sin(angle + Pi);y = 460 + 110.0f * cos(angle + Pi);glVertex3f(x, y, z);}
}
void DrawCirclet2()
{glColor3f(1.0f, 0.0f, 0.0f);GLfloat x, y, z, angle;z = 0.0f;glColor3f(1.0, 1.0, 1.0); glBegin(GL_LINES);for (angle = 0.0f; angle <= Pi; angle += (Pi / 1000000.0f)){x = 610 + 170.0f * sin(angle);y = 170 + 200.0f * cos(angle);glVertex3f(x, y, z);x = 610 + 170.0f * sin(angle + Pi);y = 170 + 200.0f * cos(angle + Pi);glVertex3f(x, y, z);}glEnd();
}
void DrawCirclet3()
{glColor3f(1.0f, 0.0f, 0.0f);GLfloat x, y, z, angle;z = 0.0f;glColor3f(0.0, 0.0, 0.0); glBegin(GL_LINES);for (angle = 0.0f; angle <= Pi; angle += (Pi / 1000000.0f)){x = 580 + 10.0f * sin(angle);y = 480 + 10.0f * cos(angle);glVertex3f(x, y, z);x = 580 + 10.0f * sin(angle + Pi);y = 480 + 10.0f * cos(angle + Pi);glVertex3f(x, y, z);}glEnd();
}
void DrawCirclet4()
{glColor3f(1.0f, 0.0f, 0.0f);GLfloat x, y, z, angle;z = 0.0f;glColor3f(0.0, 0.0, 0.0); glBegin(GL_LINES);for (angle = 0.0f; angle <= Pi; angle += (Pi / 1000000.0f)){x = 640 + 10.0f * sin(angle);y = 480 + 10.0f * cos(angle);glVertex3f(x, y, z);x = 640 + 10.0f * sin(angle + Pi);y = 480 + 10.0f * cos(angle + Pi);glVertex3f(x, y, z);}glEnd();
}
void display(void)
{glClear(GL_COLOR_BUFFER_BIT);glBegin(GL_LINE_LOOP);glColor3f(1.0f, 0.2f, 0.2f);glVertex3f(50, 50, 0.0);glVertex3f(75, 60, 0.0);glVertex3f(100, 50, 0.0);glVertex3f(90, 75, 0.0);glVertex3f(100, 100, 0.0);glVertex3f(75, 90, 0.0);glVertex3f(50, 100, 0.0);glVertex3f(60, 75, 0.0);glEnd();glColor3f(1.0f, 1.0f, 0.0f);glRectf(295.0f, 350.0f, 305.0f, 150.0f);glColor3f(1.0f, 0.2f, 0.2f);q = 5;m = 300;p = 400;for (int i = 1; i < 11; i++){q = q + 5;DrawCircle();}glColor3f(1.0f, 1.0f, 1.0f);DrawCirclet1();DrawCirclet2();DrawCirclet3();DrawCirclet4();glFlush();glBegin(GL_TRIANGLES);glColor3f(1.0f, 0.0f, 0.0f);glVertex2f(620.0f, 420.0f);glVertex2f(570.0f, 420.0f);glVertex2f(610.0f, 440.0f);glEnd();glFlush();}
void reshape(int width, int height)
{glViewport(0, 0, width, height);glMatrixMode(GL_PROJECTION);glLoadIdentity();gluOrtho2D(0.0, width, 0.0, height);
}
void keyboard(unsigned char key, int x, int y)
{
}
int main(int argc, char** argv)
{glutInit(&argc, argv);glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);glutInitWindowSize(900, 600); glutInitWindowPosition(230, 80);glutCreateWindow("Basic");init();glutDisplayFunc(display);glutReshapeFunc(reshape);glutKeyboardFunc(keyboard);glutMainLoop();return 0;
}